Django Background Tasks
I started working in django a while ago and I am in love with it. Everything is just too simple unlike Nodejs where the callbacks suck the life out of you.
You can find countless tutorials online to get started with this excellent framework . I would highly recommend codingforentrepreneurs .Do watch their tryDjango series . Here is a link to their YouTube channel.
Now lets come back to the point. A few days back I wanted to run some background tasks in Django . I had no idea where to get started . Google came to my rescue . The answers I found were :
- Celery
- Threading
- Redis Queue
- Django-Channels
- Twisted
And many more… But all I wanted to do was some background work. No! I am not afraid to learn something new , but to install a whole new bulky framework for such a small task seemed like an overhead to me .Secondly I am a Windows user . And most of these frameworks were deigned for Linux based systems. Don’t believe me ??? See it for yourself .
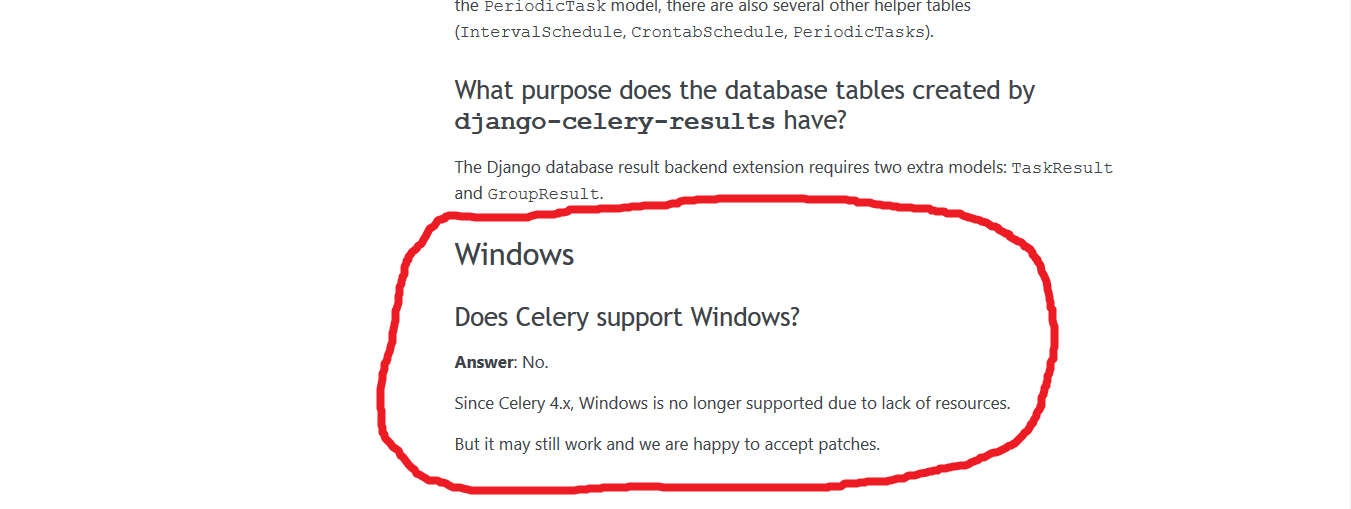
So what next ?
This is where django-background-tasks comes in . Just like many of these frameworks, it too is a python package .Here is the link to their documentation.
Here we will create a demo django project project called background .
Open your command prompt in Windows . Head to whichever directory you want and create a folder background .
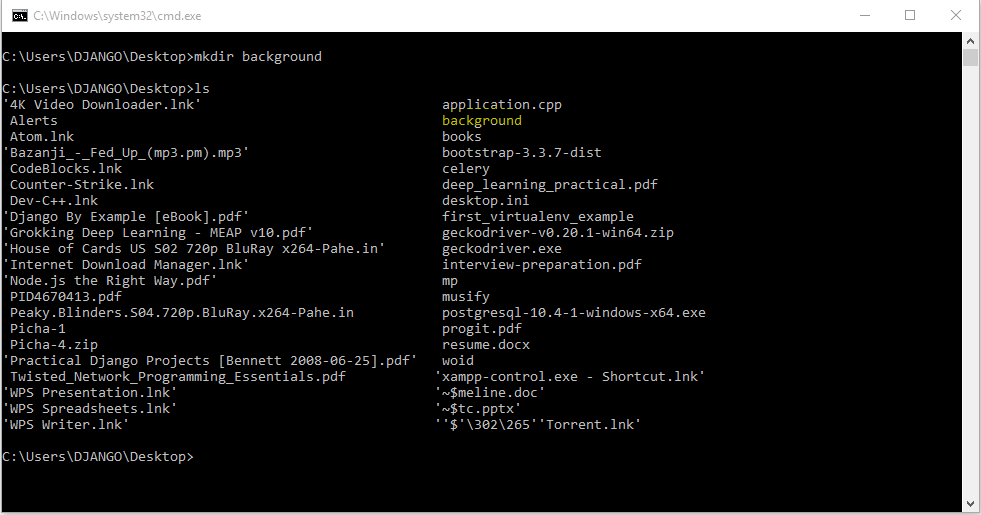
Next , inside the background the directory you need to activate a virtual environment . I am using python 2.7 .

The dot in the end signifies that the virtual environment needs to be created here . This will install pip and other dependencies.
Next activate the virtual environment .
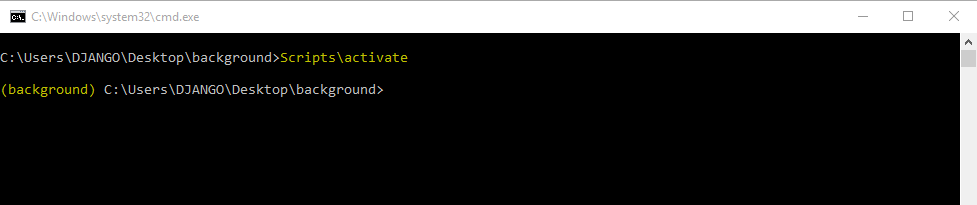
You will see ( background ) in the beginning of the command prompt .
Now lets install django and django-background tasks.


Now lets start our project
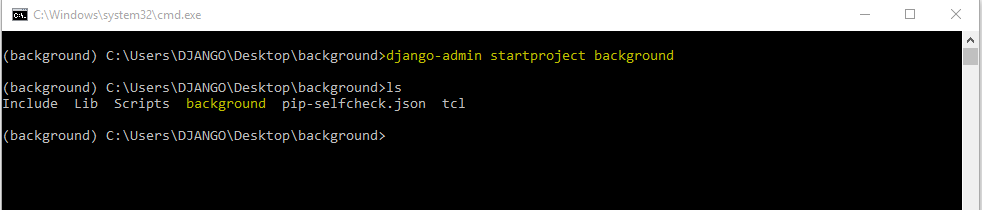
Move into the background directory (the one which is highlighted in the previous image ).
Add this line inside your settings.py .

Let us now start our app . We will call it background_app .

Add this to your INSTALLED_APPS in settings.py .
If you have followed along ,
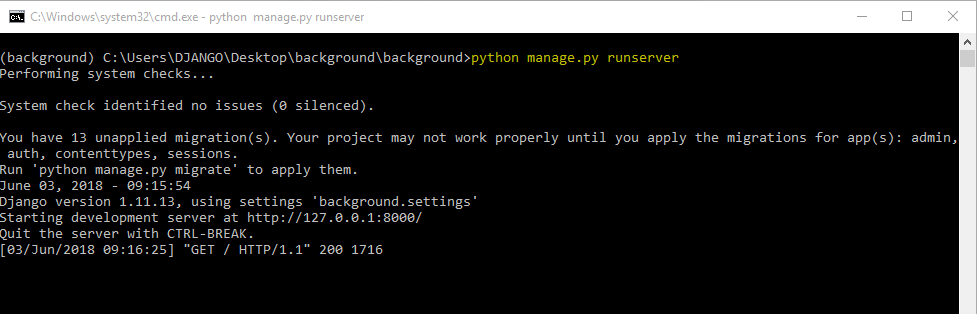

you can start the server to just check everything is working fine.
Now lets add our background tasks .
Let us create a view inside our background_app called background_view.
Make appropriate changes to the urls.py . Also create a urls.py inside your app .
This is how everything should look like
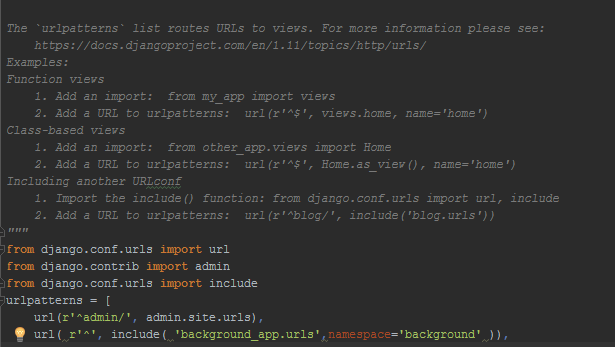

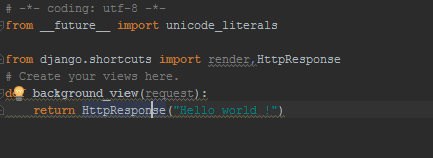
And before running the server type in
python manage.py migrate
If you run the server

Let us say that every time I go to my homepage I want to print Hello World on the console . Here is the code for the same :
Now before you go to the page there is one more thing you need to do .
Open a new terminal and head to the same path of you project and activate your virtual environment. Next , type in
python manage.py process_tasks
Now if I go to my home page

So how does this happen , you ask ?
First we import background from background_task .
Next we define a function hello which we want to fire when the user visits our homepage .
We need to initialize it with the background decorator which takes in a number as its argument .This number denotes the value in seconds as to how long after the function is called should it actually execute. Here 5 means 5 seconds . Nothing changes even if your function takes in any arguments.
Also if you go to your admin page( create a superuser first ) this is what you will see

A list of scheduled and completed tasks.
If you open these , you will find Tasks to be empty and Completed tasks having one entry.
That is fine but what if I want to run a task periodically . Django-background-tasks has a solution for that as well .
When calling the function just one more thing
repeat=10 denotes the interval after which you want to execute the task.
You can also add repeat_until = None to run the task indefinitely.
And again ,if you want to add any parameters to the function they must be passed first . As the documentation says

Here user.id is the parameter the function takes.
But this will fire the function every time we visit our homepage . What if I want to start executing it as soon as my server is up and running .
Go to your main urls.py and call the function from there .
You can add multiple tasks here ie call multiple functions one after another .
However this may get you in trouble with sqlite. You may get
django.db.utils.OperationalError: database is locked
when using SQLite.
As the documentation says:
This is a SQLite specific error, see https://docs.djangoproject.com/en/dev/ref/databases/#database-is-locked-errors for more details.
In case you get this error you will have to migrate from sqlite to some other db. I personally like PostgreSQL mainly because it is simple . Here is a great tutorial for the same .
Just remember , create the database with the username postgres and the password you provided during installation . And replace this

with

in your main settings.py .
Thanks for your valuable time .
I bid you farewell .
Comments
Post a Comment